Yet Another Unit Test Library
Tiny Test is a minimal unit test library for C/C++. It is designed especially for embedded systems keeping in mind the limitation and capabilities of the platform. This library also supports Arduino environment.
- Low memory usage
- Supports a wide range of compilers and processor architectures
- Arduino compatible
- Color debug output
- Very easy to use
How to use
- Add Test and Assert Result :
1 2 3 4
ADD_TINY_TEST(test_0){ int i = rand()%50; ASSERT_TEST_RESULT(i != 1); }
- Run Test :
1
RUN_TINY_TEST(test_0);
- Add Test Suit :
1 2 3
ADD_TEST_SUITE(test_suit){ RUN_TINY_TEST(test_0); }
- Run Test Suit :
1
RUN_TEST_SUITE(test_suit);
- Generate Test Report :
1
TINY_TEST_REPORT();
Arduino Example
- Example code for Arduino :
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47
#include "tinytest.h" ADD_TINY_TEST(test_0){ int i = rand()%50; ASSERT_TEST_RESULT(i != 1); } ADD_TINY_TEST(test_1){ int i = rand()%50; ASSERT_TEST_RESULT(i == 2); } ADD_TINY_TEST(test_2){ int i = rand()%50; ASSERT_TEST_RESULT(i != 3); } ADD_TEST_SUITE(test_suit){ RUN_TINY_TEST(test_0); RUN_TINY_TEST(test_1); RUN_TINY_TEST(test_2); } int popup(const char * input){ if(!strncmp(input, "Y", strlen("Y"))){ DEBUG_DIVIDER("*", TEST_DIVIDER_LENGTH); DEBUG_OK("Tiny Test Starting"); RUN_TEST_SUITE(test_suit); TINY_TEST_REPORT(); DEBUG_INPUT(NULL, "Press Enter To Exit", 0); return 1; } return 0; } void setup(){ SET_CLOCK_SOURCE(&millis); ARDUINO_ONLY(Serial.begin(115200)); ATTACH_DEBUG_STREAM(&Serial); DEBUG_DIVIDER("*", TEST_DIVIDER_LENGTH); DEBUG_INPUT(&popup, "Run Test? (Y/N)", 0); DEBUG_OK("Going To Loop"); } void loop(){ // Do whatever you want }
Sample Output
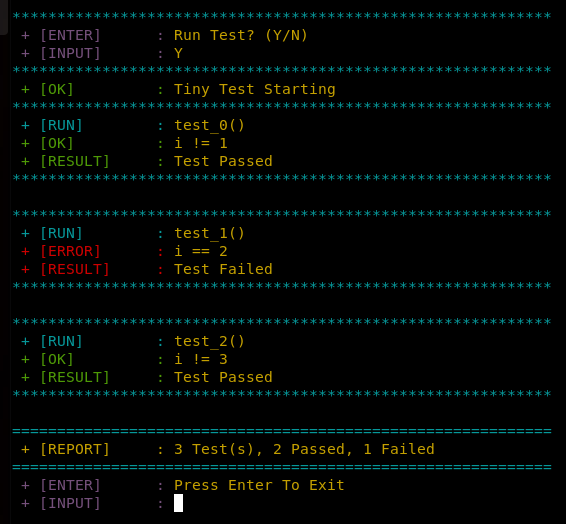