Intro
A highly configurable & lightweight debug library that prints debug messages beautifully. It works in Node.js and Web browser environments with very low memory footprint.
Features
- Beautifully colored and formatted
- Pretty prints current file name, function and and line number
- Pretty prints date, time with formatting
- Attach multiple streams and pipe the debug outputs
- Node process and System process health check
- Schedule memory monitor
- Alarm on crossing memory thresholds
- System memory high watermark
- Highly configurable
- Very low memory footprint
- No third party library dependencies
Screenshot
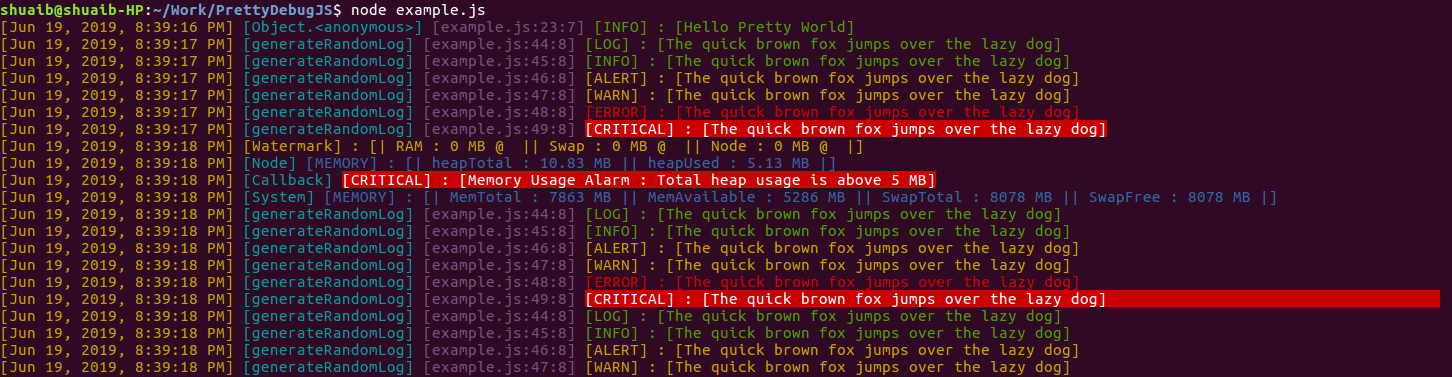
Install
$ npm install pretty-debug
Usage
Pretty Debug exposes a debug object. Just attach any stream you want to the module to pipe debug messages. process.stdout is the default steam which prints output to console. Any other streams like TCP socket or request to an HTTP server can be attached on runtime to pipe the debug output.
API Documentation
- pretty-debug
- .color :
Object.<string>
- .setOptions(userOptions)
- .generatePolicy(lowerLimit, upperLimit) ⇒
Object.<policy>
- .attachStream(stream)
- .detachStream(stream)
- .log(...var_args)
- .info(...var_args)
- .alert(...var_args)
- .warn(...var_args)
- .error(...var_args)
- .critical(...var_args)
- .nodeMemoryMonitor(stream, callback)
- .sysMemoryMonitor(alarmPolicy, callback)
- .memoryWatermark()
- .scheduleHealthCheck(inputFunc, timeInMinutes)
- .color :
For detail information, please see API Documentation.
Basic usage
-
Create debug instance :
const debug = require('pretty-debug');
-
Set options :
debug.setOptions({ enable: true, // Turning on debug print enableColor: true, // Enabling color output enableGC: true, // Enabling autometic gerbage collection debugLevel: 6, // Setting debug level 6 (Upto INFO) } });
-
Attach stream :
debug.attachStream(sock); // Attaching new stream to serve debug messages
-
Detach stream :
debug.detachStream(sock); // Detaching stream
-
Print in different debug levels :
debug.log('The quick brown fox jumps over the lazy dog'); debug.info('The quick brown fox jumps over the lazy dog'); debug.alert('The quick brown fox jumps over the lazy dog'); debug.warn('The quick brown fox jumps over the lazy dog'); debug.error('The quick brown fox jumps over the lazy dog'); debug.critical('The quick brown fox jumps over the lazy dog');
-
Show highest RAM usage :
debug.memoryWatermark(); // Shows highest RAM usage in MB.
-
Show memory used by OS :
debug.sysMemoryMonitor(); // Shows memory information of operating system
-
Show memory used by Node.js :
debug.nodeMemoryMonitor((); // Shows memory information of Node.js system
-
Set alarm policy for RAM usage :
debug.nodeMemoryMonitor({ heapTotal: { upperLimit : 5 } // Trigger alarm when heap usage is greater than 5 MB }, function(){ debug.critical('Memory Usage Alarm : Total heap usage is above 5 MB'); // Do other things like send email! });
Example
In this example a debug instance is created. For multiple stream demonstration, a TCP server is created. TCP socket is attached to the debug module. So when a client connects to that TCP server, color debug output will be shown on the client console. Health check scheduler is also demonstrated in this example.
const net = require('net');
const debug = require('pretty-debug');
var PORT = 6969;
var HOST = '0.0.0.0';
debug.setOptions({
nodeMemoryMonitor:{
fields: {
rss: false,
external: false
}
}
});
debug.info('Hello Pretty World');
debug.scheduleHealthCheck(function(){
debug.memoryWatermark();
debug.sysMemoryMonitor();
debug.nodeMemoryMonitor({
heapTotal: { upperLimit : 5 }
}, function(){
debug.critical('Memory Usage Alarm : Total heap usage is above 5 MB');
// Do other things like send email!
});
}, .02);
net.createServer(function(sock){
debug.attachStream(sock);
sock.on('end', function() {
debug.detachStream(sock);
});
}).listen(PORT, HOST);
function generateRandomLog(){
debug.log('The quick brown fox jumps over the lazy dog');
debug.info('The quick brown fox jumps over the lazy dog');
debug.alert('The quick brown fox jumps over the lazy dog');
debug.warn('The quick brown fox jumps over the lazy dog');
debug.error('The quick brown fox jumps over the lazy dog');
debug.critical('The quick brown fox jumps over the lazy dog');
}
setInterval(function(){
generateRandomLog();
}, 678)
Run Example
To run the example above, simply put this command and press Enter!
$ node Examples/example.js
Sample Output
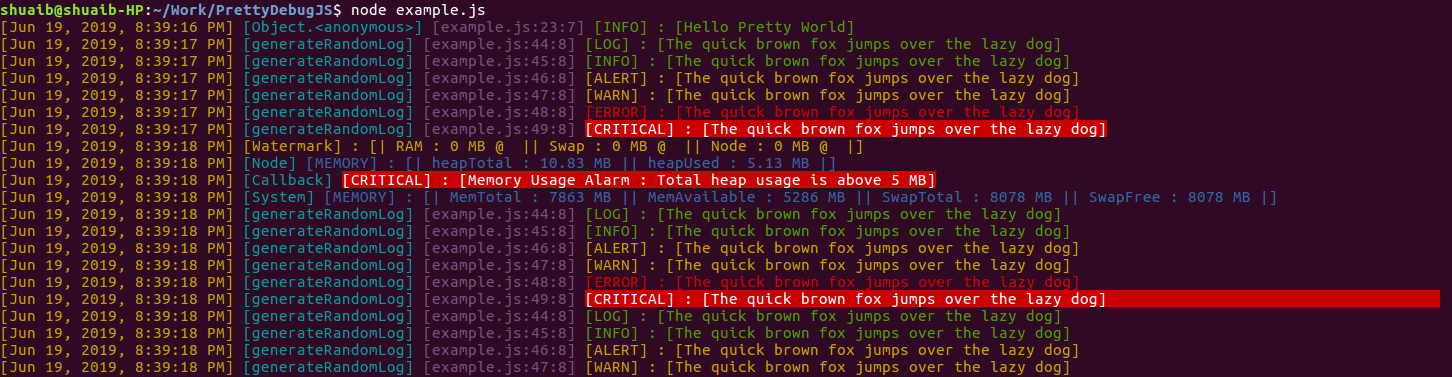
Tutorials
All the tutorials and examples can be found in the link below :
- How To Use
- Basics
- Memory Monitor
- Shedule Healthcheck
- Set Alarm
- Set Options
- Attach Multiple Stream
- Send Email On Alarm
- Summary
Customization
As it was mentioned earlier, this library is highly configurable. For more details, please see Default Options.
Contributing
If you are interested in requesting a feature, fixing issues and contributing directly to the code base, please do not hesitate.
License
Licensed under the MIT License.